思考并回答以下问题:
- Delegate和delegate的区别是什么?public abstract Delegate Action {get;set;}是什么意思?
- internal和public的区别是什么?哪个范围更大?
- IEnumerator里面基本都是while(true)。怎么理解?
- 每个a.json文件都对应一个a.cs实体数据文件。暗黑战神每个xml对应一个数据实体cs文件,都是GameData的子类。然后还有一个GameDataController类来管理所有这些GameData的子类。怎么理解?
- 静态构造函数主要目的是用于初始化一些静态的变量。怎么理解?
- 基本没有脚本是预先挂载的,都是动态挂载。
- 扩展方法第一个参数前缀为this,表示需要扩展类对象,从第二个参数开始,为扩展方法参数列表。怎么理解?
- default(T)是什么意思?为什么要这样写?if(default(T) == null)是判断什么的?
资源加载
Unity3d常用的两种加载资源方案:Resources.Load和AssetBundle。
1、Resources.Load:使用这种方式加载资源,首先需要在Asset目录下创建一个名为Resources的文件夹,这个命名是U3D规定的方式,然后把资源文件放进去,当然也可以在Resources中再创建子文件夹,当然在代码加载时需要添加相应的资源路径。
2、项目中更常用的是使用AssetBundle的方式动态加载游戏对象。
使用AssetBundle打包预设或者场景可以将与其相关的所有资源打包,这样很好地解决资源的依赖问题,使得我们可以方便的加载GameObject。
\client\Assets\Scripts\ClientCore\Resource.cs
1 | using System; |
client\Assets\Scripts\ClientCore\ResourceManager.cs
1 | using System; |
游戏初始化
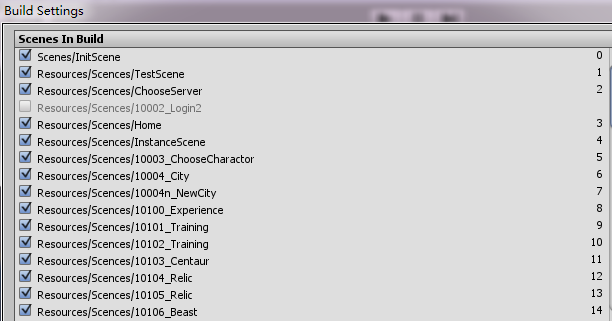
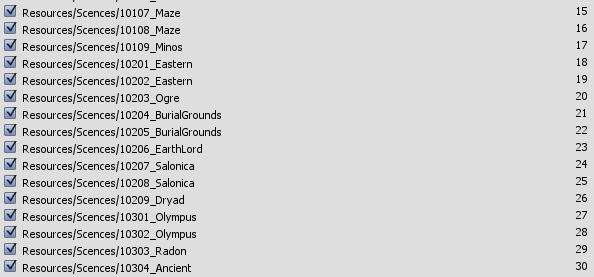
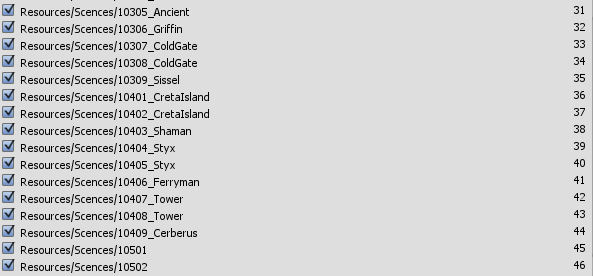
最开始的是InitScene,只有这个场景在Scenes文件下,其他都在Resources文件夹下。这个场景只有一个Driver空对象,带有Initializer脚本。这个脚本放在Plugins文件夹下。
\client\Assets\Plugins\Init\Initializer.cs
1 | using UnityEngine; |
\client\MogoSolution\LoaderLib\Init\PluginCallback.cs
1 |
\client\Assets\Plugins\Init\Driver.cs
1 |
|
配置文件
XML
暗黑战神\client\Assets\Resources\data\xml\FXData.xml
1 | <root> |
MogoSolution\GameData\GameData.cs
1 |
|
暗黑战神\client\MogoSolution\GameData\FXData.cs
1 |
|
使用FXData
1、FXData.dataMap.ContainsKey(hitFx)
2、Linq1
FXData.dataMap.Values.Where(t => t.player.Contains(playerName) && !String.IsNullOrEmpty(t.resourcePath) && !t.resourcePath.Contains(weaponType)).Select(t => t.resourcePath)
1 | var fx = FXData.dataMap.Get(id); |
MogoSolution
\client\MogoSolution\LoaderLib\Utils\SystemConfig.cs
1 |
|
MogoSolution\Common\Utils\MecanimEvent.cs
1 |
|
\MogoSolution\LoaderLib\Utils\Timer\TimerData.cs
1 | using System; |
TimerHeap.cs heap堆
1 |
|
Actors
Actor开头的脚本都是挂载到游戏对象上的。
\Actors\ActorParent.cs 需要继承MonoBehavior
1 | // Actor对象的基类 |
\client\Assets\Scripts\Actors\ActorPlayer.cs
1 | using UnityEngine; |
\client\Assets\Scripts\Actors\ActorDummy.cs 纯客户端的怪物
1 | using UnityEngine; |
\Actors\ActorBullet.cs
1 | // 飞行物控制器(用于火球,弓箭等物体的特效播放,追踪目标的显示逻辑 |
GUI
\client\Assets\Scripts\GUI\MogoFx\MogoCameraCullByLayer.cs
1 | using UnityEngine; |
GUI/Mogo/UILogicManager.cs UI逻辑绑定管理
1 | using Mogo.Util; |
GameLogic
\client\Assets\Scripts\GameLogic\IEventManager.cs
1 |
|
EntityParent.cs 客户端Entity基类,这是个partial类,方法在这里,属性在ParentProperty.cs里
1 | using UnityEngine; |
\client\Assets\Scripts\GameLogic\Entities\ParentPartial\ParentProperty.cs
1 | using UnityEngine; |
\client\Assets\Scripts\GameLogic\Entities\EntityMonster.cs 服务器端控制的怪物
1 | using UnityEngine; |
GameLogic/BattleSystem/BattleManager.cs 战斗管理系统
1 | using UnityEngine; |
GameLogic/BattleSystem/PlayerBattleManager.cs
1 | using UnityEngine; |
GameLogic\SfxSystem\SfxHandler.cs
1 |
|
\client\Assets\Resources\data\xml\SkillBuff.xml
1 |
|
\client\MogoSolution\GameData\SkillBuffData.cs
1 | /*---------------------------------------------------------------- |
\client\Assets\Scripts\GameLogic\BattleSystem\BuffManager.cs
1 | using UnityEngine; |
Gears
暗黑战神\client\Assets\Scripts\Gears\GearParent\GearParent.cs
1 | /*---------------------------------------------------------------- |
暗黑战神\client\Assets\Scripts\Gears\DropRock\DropRock.cs 丢石头机关
1 | using UnityEngine; |
MogoWorld
暗黑战神\client\Assets\Scripts\GameLogic\MogoWorld.cs
1 | /*---------------------------------------------------------------- |
EntityMyself
\client\Assets\Scripts\GameLogic\Entities\EntityMyself.cs 玩家控制的角色的逻辑控制类
1 | /*---------------------------------------------------------------- |
场景管理
场景自带了fog,然后light是disable的,没有摄像机。
\client\Assets\Resources\data\xml\GlobalData.xml
1 | <root> |
\client\MogoSolution\GameData\GlobalData.cs
1 | using System; |
client\Assets\Resources\data\xml\map_setting.xml
1 |
|
\client\MogoSolution\GameData\MapData.cs
1 | using System; |
\client\Assets\Scripts\GameLogic\ScenesManager.cs
1 |
|
AssetCacheMgr
\client\MogoSolution\LoaderLib\Utils\AssetCacheMgr.cs
1 | using UnityEngine; |
任务与剧情
\client\Assets\Resources\data\xml\ChineseData.xml
1 |
|
client\MogoSolution\GameData\LanguageData.cs
1 | /*---------------------------------------------------------------- |
client\Assets\Resources\data\xml\TaskData.xml
1 |
|
\client\MogoSolution\GameData\TaskData.cs
1 | /*---------------------------------------------------------------- |
\client\Assets\Scripts\GameLogic\TaskSystem\TaskManager.cs
1 | /*---------------------------------------------------------------- |
CG
\client\Assets\Resources\data\xml\StoryCG1.xml
1 |
|
\client\Assets\Scripts\GameLogic\StorySystem\StoryManager.cs CG故事管理
1 | using System; |
NPC
client\Assets\Resources\data\xml\NPCData.xml
1 |
|
\client\Assets\Resources\data\xml\NPCData.xml
1 | /*---------------------------------------------------------------- |
\client\Assets\Scripts\Actors\ActorNPC.cs
1 | using UnityEngine; |
\client\Assets\Scripts\GUI\Mogo\MogoObjOptWorker.cs
1 | using UnityEngine; |
\client\Assets\Scripts\GUI\Mogo\MogoObjOpt.cs
1 | using UnityEngine; |
\client\Assets\Scripts\GameLogic\Entities\EntityNPC.cs NPC的逻辑控制
1 | using UnityEngine; |
\client\Assets\Scripts\AvatarControl\MogoMotorNPC.cs
1 | using UnityEngine; |
PathPoint
\client\Assets\Plugins\Utils\PathPoint.cs
1 | /*---------------------------------------------------------------- |
\client\Assets\Plugins\Utils\SubPathPoint.cs
1 | using UnityEngine; |
新手引导
\client\Assets\Resources\data\xml\guide.xml
1 |
|
\client\MogoSolution\GameData\GuideXMLData.cs
1 | using System.Collections; |
\client\Assets\Scripts\GameLogic\GuideSystem\GuideSystem.cs 新手指引系统
1 | using System; |
Utils
\client\Assets\Scripts\Utils\MogoUtils.cs 游戏逻辑相关通用工具类
1 | using System.Collections.Generic; |
SkillManager
\client\Assets\Scripts\GameLogic\Enum\Constants.cs
1 | using System.Collections.Generic; |
\client\Assets\Resources\data\xml\SkillAction.xml
1 |
|
\client\MogoSolution\GameData\SkillAction.cs
1 | using System; |
\client\Assets\Scripts\GameLogic\SkillSystem\SkillManager.cs 技能管理系统
1 | using UnityEngine; |
LuaTable
\client\MogoSolution\LoaderLib\Utils\LuaTable.cs
1 | using System; |