思考并回答以下问题:
- Func()委托的第几个参数是返回值?为什么又自定义自己的MyFunc()委托?怎么定义?
- 对象池为什么使用Queue数据结构?
设计图
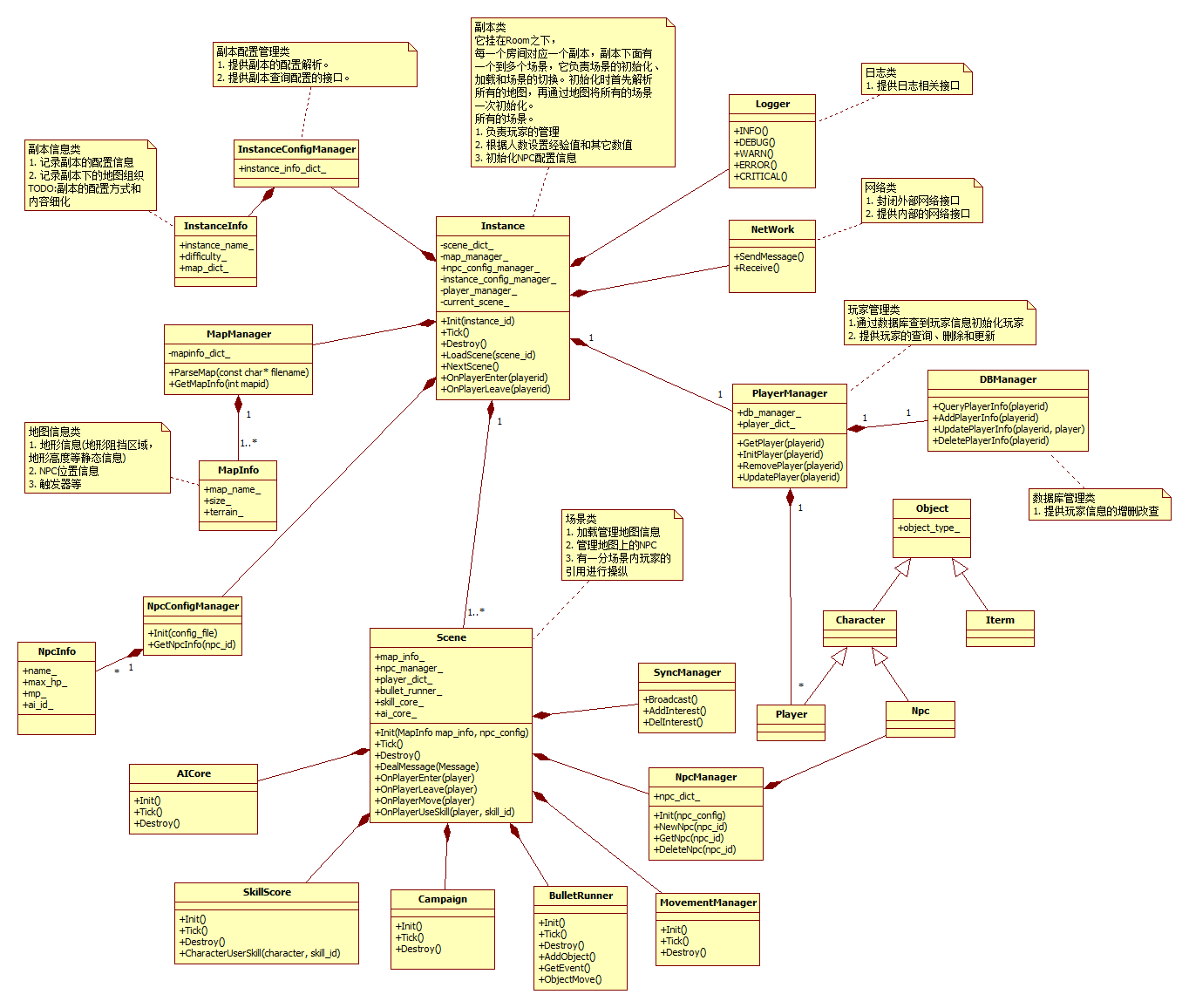
Util
\Public\Common\Util\MyActionAndFunc.cs
1 | using System; |
\Public\Common\Util\ObjectPool.cs
1 | using System; |
Triggers
\Public\GfxModule\Skill\Trigers\ColliderScript.cs
1 | using UnityEngine; |
\Public\GfxModule\Skill\Trigers\TriggerUtil.cs
1 | using System; |
\Public\GfxModule\Skill\Trigers\ColliderTriggerUtility.cs 把ColliderScript组件添加到对象
1 | using System; |
SkillSystem
\Public\SkillSystem\ISkillTriger.cs 技能触发器
1 | using System; |
\Public\SkillSystem\SkillInstance.cs 技能实例
1 | using System; |
\Public\SkillSystem\ISkillTrigerFactory.cs 工厂
1 | using System; |
\Public\SkillSystem\SkillConfigManager.cs 技能配置管理
1 | using System; |
Internal
\Public\GfxLogicBridge\Internal\ResourceManager.cs
1 | using System; |
AISystem
\Public\GameObjects\AiCommand\IAiCommand.cs
1 | using System; |
\Public\AiSystem\AbstractAiCommand.cs
1 | using System; |
GameObjects
\Public\GameObjects\NpcManager.cs
1 | using System; |
GfxLogicBridge
\Public\GfxLogicBridge\SharedData\InvokeArgs.cs
1 | using System; |