思考并回答以下问题:
- 为什么模块的入口就用单例模式?
- 坚持一个类不超过200行,慢慢下去就会有架构思想了。
- 时序图和类图太重要了,时序图还重要一点,怎么理解?
- 为什么要保证UIManager最先执行?怎么设置?
实现
消息交互无非两种方式:
1、引用
2、委托
思路
以Panel为单位。C层管理所有的子控件,控件之间互相交互通过C层(中介者模式)完成,降低耦合。C层怎么找到子控件?用GameObject.Find()是深度遍历,比较麻烦。增加一个UIManager,Panel与Panel之间通过UIManager进行通信。然后让子控件主动上报给UIManager,Panel可以从UIManager取得控件。
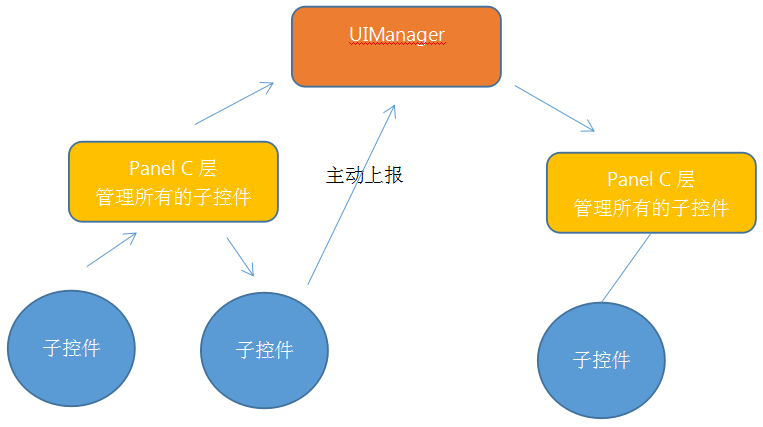
如何主动上报?
- 1、给任何子控件挂一个万能的UIBehaviour。
- 2、UIBehaviour将自己注册进UIManager。
UIManager:
1、怎么样保存注册到UIManager里面的子控件?
以Panel为单位划分,每个Panel下面又管理了很多子控件。
UIManager.cs 模块管理者
1 | using System.Collections; |
UIBase.cs 所有Panel,C层基类,挂载到Panel上
1 | using System.Collections; |
UIBehaviour.cs 挂载到需要注册的子控件上,比如一个Button,显示的text就不要挂载了
1 | using System.Collections; |
实例
先把UIManager设置为最先执行。
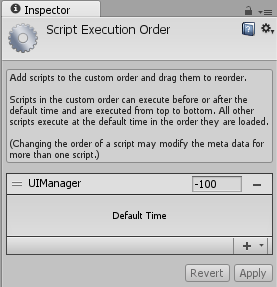
创建一个空对象UIManager,挂载UIManager.cs
创建一个LoadingPanel面板,挂载LoadingCtrl脚本
LoadingCtrl.cs 挂载到LoadingPanel上
1 | using System.Collections; |
RegCtrl.cs 挂载到RegPanel上,以Panel为单位,所以下面可以有一个Button_N
1 | using System.Collections; |
注意事项:
- 1、在同一个Panel里面,要使用的控件名字不能一样。
- 2、不同的Panel的名字也不能一样。
- 3、不同的Panel下面的子控件的名字可以一样。