思考并回答以下问题:
- 以下代码自己实现一遍。
添加摄像机
新建一个名为ThirdPersonCam的脚本。代码如下:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27using UnityEngine;
using System.Collections;
public class ThirdPersonCam : MonoBehaviour
{
// 摄像机所跟随的对象
public Transform follow;
// 摄像机在水平方向与对象的距离
public float distanceAway;
// 摄像机在垂直方向与对象的距离
public float distanceUp;
// 过渡速度
public float smooth;
// 摄像机的目标速度
private Vector3 targetPosition;
// 在LateUpdate中执行摄像机操作,确保在对象的操作完成之后
void LateUpdate ()
{
// 计算目标位置
targetPosition = follow.position + Vector3.up * distanceUp - follow.forward * distanceAway;
// 对当前位置进行插值计算
transform.position = Vector3.Lerp(transform.position, targetPosition, Time.deltaTime * smooth);
// 使摄像机观察对象
transform.LookAt(follow);
}
}
将参数进行如下设置。
- Follow:设置为player游戏对象。
- Distance Away:设置为5。
- Distance Up:设置为2。
- Smooth:设置为3。
1 | using UnityEngine; |
反向动力学
当开启iKPass后,会在每一帧里调用MonoBehaviour的OnAnimatorIK()函数。IK的处理也只能在OnAnimatorIK()函数里。
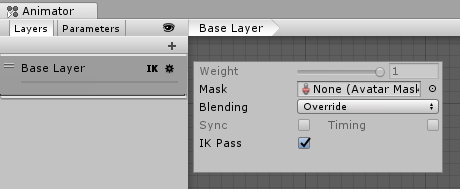
角色上绑定的IKCtrl脚本代码如下:
1 | using UnityEngine; |
需要注意Unity中IK的限制,AvatarIKGoal也就是IK的目标只有RightHand、LeftHand、RightFoot和LeftFoot这4种,而且IK是某个身体部分(比如右臂)的反向动力学,并不是全身的。