思考并回答以下问题:
- MVC的目的是为了解耦合,是怎么实现解耦合的?
- MVC的一层可以省略吗?
MVC
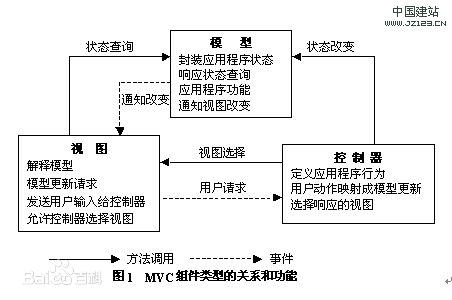
虚线是间接调用,进一步的解耦合的话可以把实线都变成虚线。
PureMVC
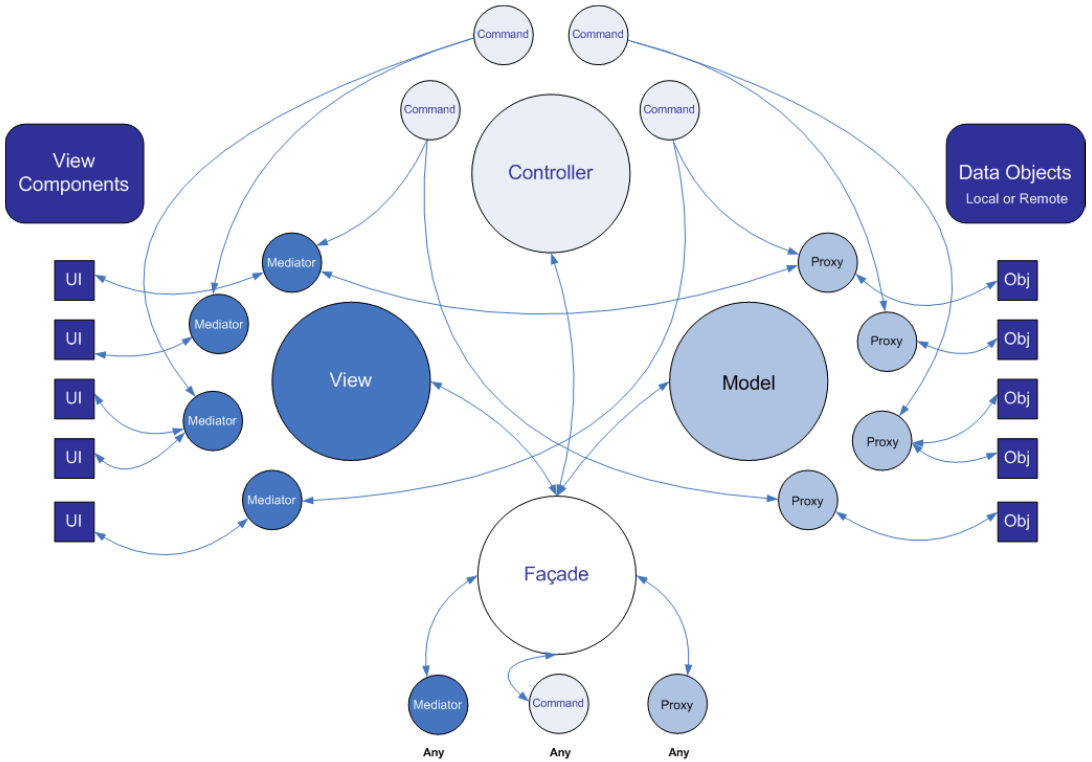
PureMVC全部是间接引用。
Controller都是命令对象。
View既要接收消息,也要发送消息。
Proxy和Mediator都需要定义本类名称。
应用
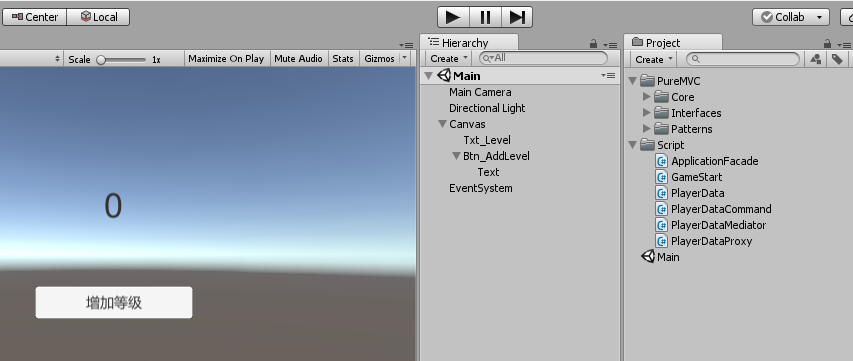
Model
PlayerData.cs
1 | using System.Collections; |
PlayerDataProxy.cs
1 | using System.Collections; |
View
PlayerDataMediator.cs
1 | using System.Collections; |
Controller
PlayerDataCommand.cs
1 | using PureMVC.Patterns; |
Facade
ApplicationFacade.cs
1 | using PureMVC.Patterns; |
客户端
GameStart.cs 挂载到Canvas上
1 | using System.Collections; |
分析
C# this 构造函数
我看的中介者模式只有一个中介者,这边有多个中介者一个UIPanel就是一个中介者里面的各种Text、Button都是使用这个中介者的对象
代理 中介者 命令 通过观察者模式进行通信 对客户端使用外观模式进行调用
控制器的命令模式把模型层的方法封装了 控制器是命令对象 客户端通过外观模式访问控制器间接调用模型
模型与视图 通过 观察者模式进行通信
每一个View都是中介者
观察者触发命令
观察者触发 使用代理模式的 命令
写代码的时候 是否 应用MVC 其实是否有解耦合的思想
之前省略了一层并不是知道原因 其实解耦合才是思想