思考并回答以下问题:
- 所有的加载都调用一个类来处理。这样的好处是:1、可以进行全局管理和优化,方便切换加载方式;2、代码各处都有各自的加载非常混乱,一旦修改就改动的非常多。
- Vector3.normalized和Vector3.Normalized的区别是什么?
- i<<=j与i|=j是什么意思?
快速跳转
商业源码
- 会用到很多设计模式,常见的有单例模式,外观模式与观察者模式,工厂模式等;
- 用到很多数据结构,例如Queue\
,Stack\ ,还有自定义的数据结构,MyDictionary,BetterList,Heap,LinkedListDictionary等; - 能不继承MonoBehaviour的就不继承,然后有很多Manager类,基本是封装一切;
- 类的层次和引用特别深,通常看懂一个功能需要找好几层,好几个类;
- 完全看懂需要画UML图和时序图。
配置</span>
1、配置文件格式 \Assets\Resources\Config\Data\Skilldata.xml
1 |
|
FFF555是黄色,00FF00是绿色。一个技能有一个ID。
Assets\Resources\Effect\Effect_Prefab\Role\Skill\zhanshi_xuanfengzhan.prefab特效的位置。使用了FX Maker插件。
Assets\Resources\Picture\Skill\zhanshi_xuanfengzhan.png图片的位置
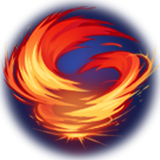
2、地址常量定义 Assets\Code\UI\Common\Const\GlobalConst.cs 实现文件的相关常量定义
1 | // 存储路径的常量 |
3、\Assets\Code\UI\Common\ReadLocclData\DataReadBase.cs 从XML读取配置的数据的基类
1 | using UnityEngine; |
\Assets\Code\Common\ConfigDataManager.cs 就是这个文件读取XML的
1 | using UnityEngine; |
\Assets\Code\UI\Skill\Model\SkillVo.cs 模型类
1 | using System; |
\UI\Common\ReadLocalData\DataReadSkill.cs
1 | using UnityEngine; |
Appear
\Code\Character\Appear\BornAppear.cs
1 | using UnityEngine; |
\Code\Character\Appear\SkillAppear.cs
1 | using UnityEngine; |
Effect
\Code\UI\Common\BillBoard.cs
1 | using UnityEngine; |
\Code\UI\Effect\FloatBloodNum.cs 浮血数字
1 | using UnityEngine; |
Scenario
\Code\UI\Task\Scenario.cs
1 | using UnityEngine; |
\Code\UI\Task\ScenarioManager.cs 剧情管理
1 | using UnityEngine; |
NewBattleSystem
NewBattleSystem/BaseBuff.cs
1 | using UnityEngine; |
Character</span>
\Character\Skill\Skill.cs
1 | using UnityEngine; |
Character\CharacterBaseProperty.cs 角色属性分离出单独类,此为属性基类。
1 | using UnityEngine; |
Code\Common\CharacterProperty.cs
1 | using UnityEngine; |
\Character\Character.cs 角色类继承MonoBehaviour,控制角色的位置,旋转,激活,面向方向
1 | using UnityEngine; |
\Character\CharacterPlayer.cs
1 | using UnityEngine; |
\Character\CharacterMonster.cs
1 | using UnityEngine; |
NPC</span>
NPC系统和Task任务系统是紧密联系着的,还有UI系统,因为需要对话。
\Assets\Resources\Config\Data\NPC.xml NPC的配置文件
1 |
|
\Assets\Code\Character\NPC\NPC.cs NPC游戏对象类,没有继承MonoBehaviour
这个类是交给NPCManager使用的,函数也是在那边调用。
1 | using UnityEngine; |
这个NPC带了一个橙色的叹号
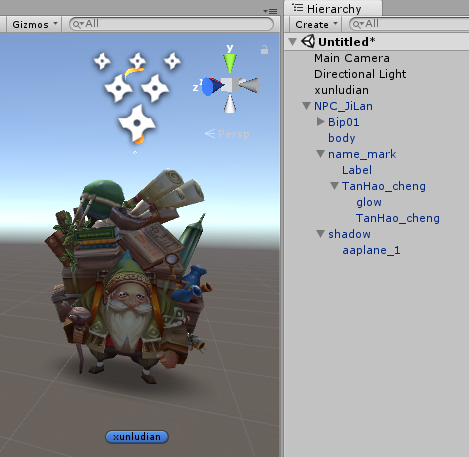
\Assets\Code\Character\NPC\NPCAction.cs NPC的行为,比如监听鼠标按下才行动,需要继承MonoBehaviour
像FindChild().GetComponent<>(),SetActice()需要用到这种的,要继承MonoBehaviour
1 | using UnityEngine; |
ui_task_dialog
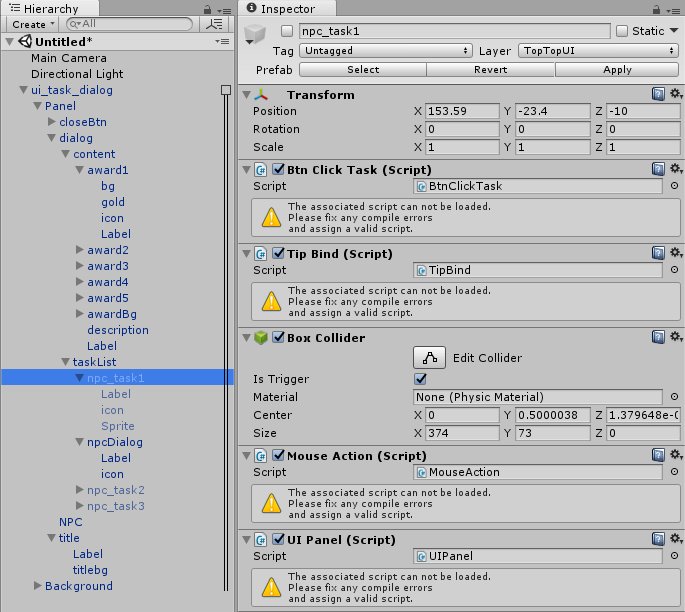
\Assets\Code\UI\Common\ReadLocclData\DataReadXml.cs 读取帮助,制作,NPC位置,任务,剧情的XML
1 | using helper; |
\Code\Character\NPC\NPCManager.cs 管理NPC对象
1 | using UnityEngine; |
Manager
\Manager\EventDispatcher.cs 定义了一堆Public委托,然后提供了执行委托的方法
1 | using UnityEngine; |
\Code\Manager\BattleManager.cs
1 | using UnityEngine; |
CDManager.cs CD管理
1 | using UnityEngine; |
\Manager\MonsterManager.cs 怪兽管理类
1 | using UnityEngine; |
Battle
\Battle\Appear.cs
1 | using System; |
JiGuan.cs
1 |
AssetBundleManager
\Code\AssetBundleManager\BundleMemManager.cs
1 | /** |
\Code\AssetBundleManager\DownloadSceneProgress.cs
1 |
Task
1 |
|
\Code\UI\Task\Task.cs
1 |