思考并回答以下问题:
大纲
- 登录注册系统
- 网络通信系统
- 角色展示系统
- 任务引导系统
- 副本战斗系统
- 强化升级系统
- 资源交易系统
- 世界聊天系统
- 任务奖励系统
码云地址:
https://gitee.com/Insister6633/DarkGod
架构
架构图
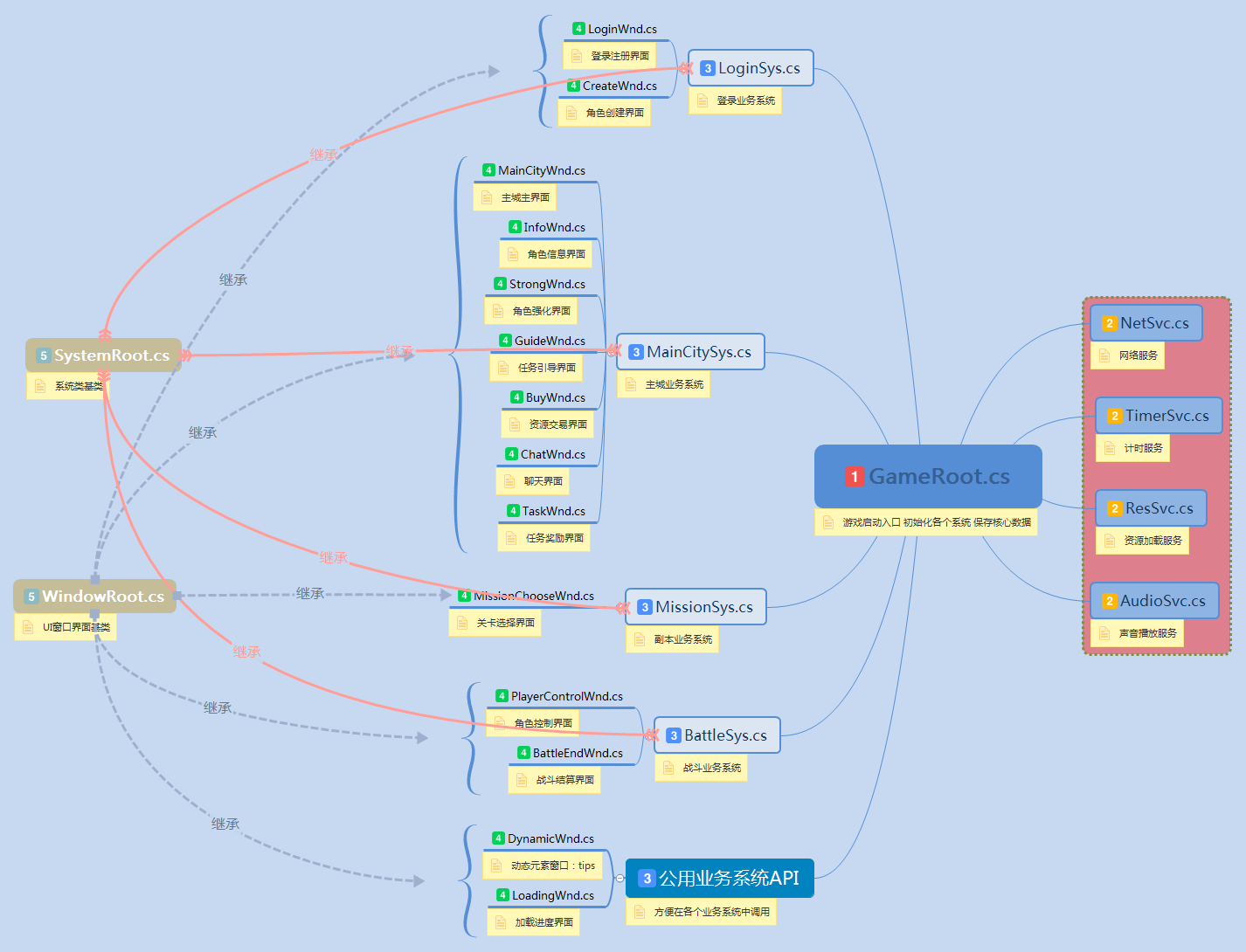
如图所示:
- 1、GamRoot是一个外观模式类,引用了各种子系统,子UI脚本;
- 2、引用分两类,一类是服务模块,一类是业务系统,业务系统都继承自SystemRoot;
- 3、业务系统管理其下的各个界面xxxWnd类,这些类都继承自WindowRoot。
脚本目录
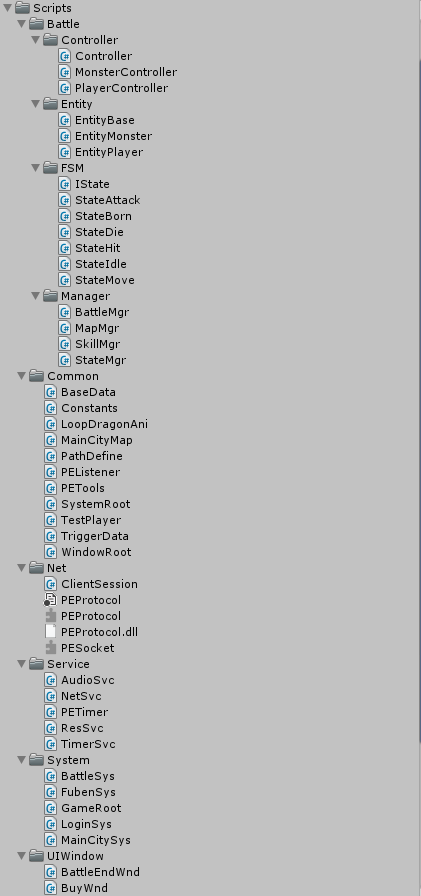
\System\GameRoot.cs 游戏启动入口
1 | using PEProtocol; |
Unity光照渲染原理
- 直接光照(Direct Lighting):光源直接照射到物体表面所以产生的光照信息。
- 间接光照(Indirect Lighting):光源照射到物体表面以后再反射到其它物体上所形成的光照信息
- 环境光(Ambient Lighting):太阳照射大气层,散射产生天空光。环境光本质是间接光照,因为散射的本质就是反射。
- 全局照明(Global Illumination):直接光照加上间接光照。
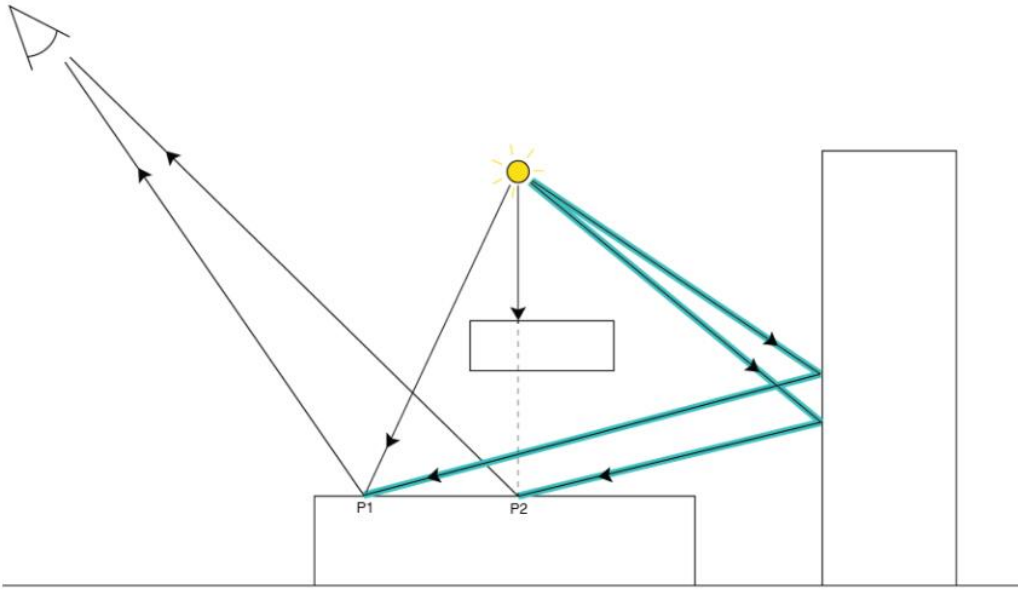
UI
\DarkGod\Client\Assets\Scripts\Common\WindowRoot.cs
1 | /**************************************************** |
Battle
Controller
\Battle\Controller\Controller.cs 表现实体控制器抽象基类
1 | using System.Collections.Generic; |
\Battle\Controller\MonsterController.cs 怪物表现实体角色控制器类
1 | using UnityEngine; |
\Battle\Controller\PlayerController.cs 主角表现实体角色控制器类
1 | using UnityEngine; |
Entity
\Battle\Entity\EntityBase.cs 逻辑实体基类
1 | using System.Collections.Generic; |
\Battle\Entity\EntityMonster.cs 怪物逻辑实体
1 | using UnityEngine; |
\Battle\Entity\EntityPlayer.cs 玩家逻辑实体
1 | using UnityEngine; |
Manager
\Battle\Manager\BattleMgr.cs 战场管理器
1 | using System; |
\Battle\Manager\MapMgr.cs 地图管理器
1 | using UnityEngine; |
\Battle\Manager\SkillMgr.cs 技能管理器
1 | using System.Collections.Generic; |
\Battle\Manager\StateMgr.cs 状态管理器
1 | using UnityEngine; |
System
\System\BattleSys.cs 战斗业务系统
1 | using PEProtocol; |